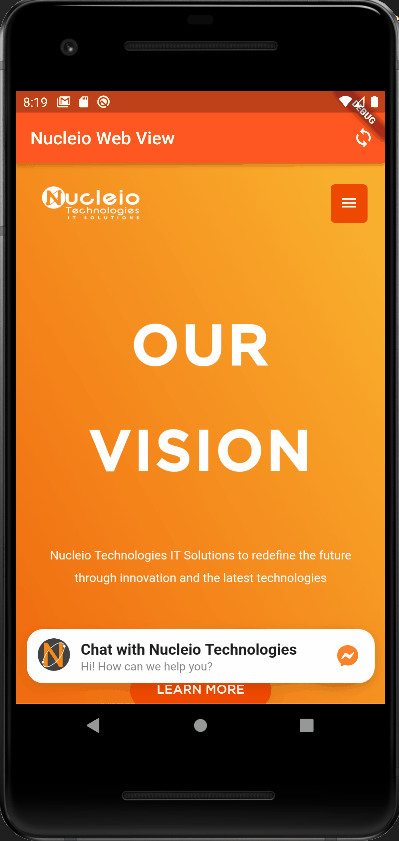
In today’s blog post, we’re going to tackle WebView and create a Flutter app project with WebView, but first, what is WebView?
Accordingly, a WebView on the Android framework was based on Blink. On the other hand, on Apple, the framework was based on WebKit. Let’s dive more into it!
WebView Origin
Blink is a clone of WebKit’s WebCore component, which was initially a fork of KDE’s KHTML and KJS libraries. It is supported by Chrome since version 28, Microsoft Edge since version 79, Opera, Vivaldi, Brave, Amazon Silk, and other Chromium-based browsers and frameworks.
Additionally, CSS vendor prefixes are similarly deprecated in the fork; existing prefixes will be phased out, and new experimental capabilities will instead be allowed on an opt-in basis.
Aside from these anticipated improvements, Blink was initially very similar to WebCore. From late 2009 until 2013, when they began work on their fork, Blink, Google was the largest contributor to the WebKit source base by contribution count from Google.
Creating the WebView Project:
WebView Dependencies
Critically, we need to set our Flutter project default SDK version to at least version 19 or higher. We can do this by going to [projectname]/android/app/build.gradle
. You should change the minSdkVersion
to 19, just like what’s shown below.
defaultConfig {
applicationId "com.example.mywebviewapp"
minSdkVersion 19 // change like this
targetSdkVersion flutter.targetSdkVersion
versionCode flutterVersionCode.toInteger()
versionName flutterVersionName
}
Now, we need the plugin called webview_flutter
, so we must execute the following command inside our Flutter project.
flutter pub add webview_flutter
WebView Project In Flutter
Finally, we can now import the webview_flutter
package into our project. To demonstrate, the code below opens the website nucleiotechnologies on WebView. You’ll notice there should be a controller existing in the code because we’ll be using it for the button on the app bar to reload the page.
Additionally, you might also notice our WebView project is very smooth and not static at all because we enabled the Javascript with its parameters set to unrestricted. Last thing, make sure to restart the whole project first because otherwise it will cause an error.
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
void main() {
runApp(
MaterialApp(
home: MyApp(),
theme: ThemeData(
appBarTheme: const AppBarTheme(backgroundColor: Colors.deepOrange),
),
),
);
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
late WebViewController? controller;
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(
title: const Text('Nucleio Web View'),
actions: [
IconButton(
onPressed: () async {
controller!.reload();
},
icon: const Icon(Icons.loop),
)
],
),
body: WebView(
initialUrl: 'https://www.nucleiotechnologies.com/',
javascriptMode: JavascriptMode.unrestricted,
onWebViewCreated: (controller) {
this.controller = controller;
},
),
);
}
WebView In Flutter / Conclusion
To conclude, we’ve tackled WebView and created a Flutter app project with WebView in which we learned that a WebView on the Android framework is based on Blink, which is a clone of WebKit’s WebCore. Finally, we set the Flutter project’s default SDK version to 19 or higher as part of the dependencies. Thank you for reading. Please share it with your friends if you find it useful.